The new three column style UISplitViewController behavior in iOS14 was giving me some headaches until I found a solution that works well for both. The code below is based on the example from Mac Catalyst > Displaying the Sidebar.
The 3 high level steps are:
- Test the user interface.
- Use the new three column style for iPad or two column style for (compact) iPhone.
- Test the split view controller style when handling transitions or segues.
Check if the user interface is an iPad and, if so, use the new three column style.
func configureHierarchy(){
if traitCollection.userInterfaceIdiom == UIUserInterfaceIdiom.pad {
print("is ipad")
configureThreeColumnSplitView()
}
else{
print("not ipad")
configureTwoColumnSplitView()
}
}
Set up the 3 view controllers: primary, supplementary, and secondary. For my Gov Job Search app, I set up a master (Search Options), supplementary (Results List), and secondary (Job Detail). Set the Class and Storyboard ID for view controller and navigation controller in the Storyboard.
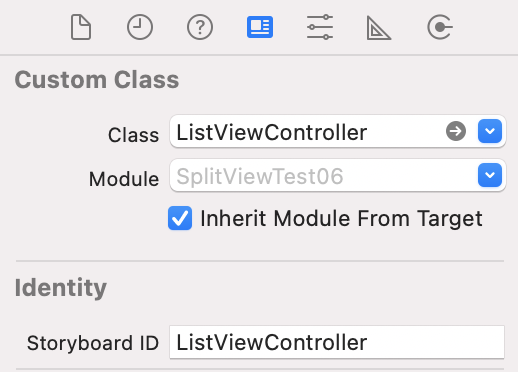
Use the new three column style for the user interface. Use the traditional two column style for the compact (iPhone) user interface.
let splitVC = SplitViewController(style: .tripleColumn)
Load the view controllers for the three column display. The navigation controllers will be added automatically for the three column display. Instantiate the navigation controllers for the two column display. Use the setViewController method to assign the primary, supplementary, and secondary view controllers.
guard let masterVC = storyboard.instantiateViewController(identifier: "MasterViewController") as? MasterViewController else {return}
splitVC.setViewController(masterVC, for: .primary)
Add conditional logic to check if the user interface uses the double or column style to handle transitions or segues accordingly. Use either the preferredDisplayMode or show method to transition between the view controllers.
if splitVC.style == .tripleColumn {
splitVC.preferredDisplayMode = .oneBesideSecondary
listViewController?.updateUI()
}else{
splitVC.show(.secondary)
}
You could use setViewController with a view controller specifically for the .compact view, however when the user rotates the device it may switch back from the compact view to the default split view. I found this Sidebar iOS 14 project helpful, but it wasn’t quite the user experience I wanted when switching back and forth with actual content on the iPhone.